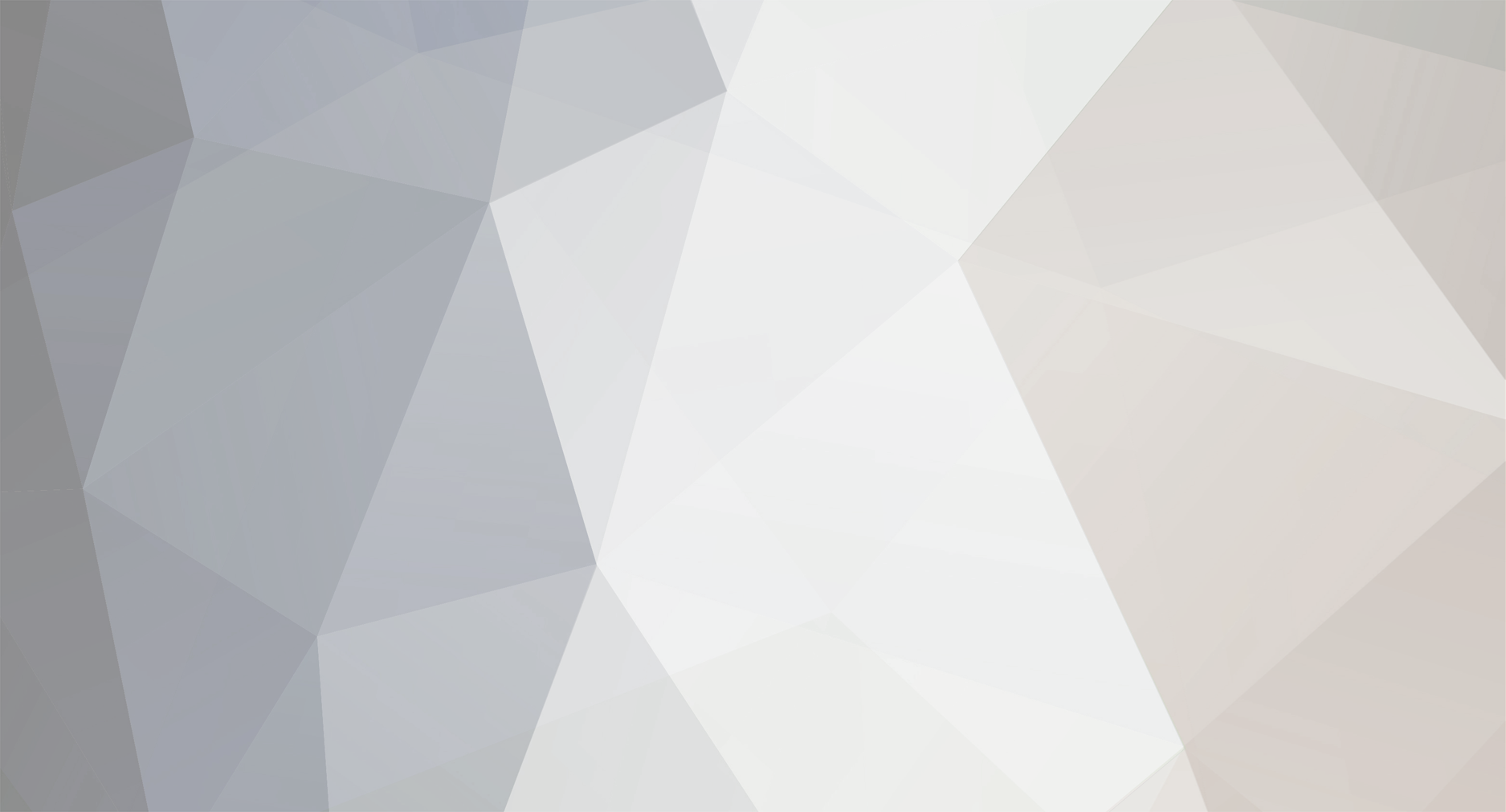
SharpEars
Premium Member-
Posts
196 -
Joined
-
Last visited
-
Days Won
5
Content Type
Profiles
Blogs
Forums
Gallery
Pipeline Tools
3D Wiki
Plugin List
Store
Downloads
Everything posted by SharpEars
-
OK, with the improved algorithm, 1,000 are possible, but it fails hard attempting 1,250: 1000 circles have been generated in 10.0 seconds Failed to create 211 of the 1250 requested after 250000 attempts Image of the results of the second of the above attempts, containing the: 1,250 - 211 = 1,039 circles that were successfully generated: I blame my algorithm's shortcomings in the last attempt on Mr. Chubby Circle, who is trying to take all of the space for himself!
-
All 750, for sure, are generated. That is the actual real count. If there is a failure to generate (i.e., max attempts exceeded at some point), I would get an error message indicating how many actually got generated. That is how I determined that 750 was pretty much the limit, at least with my prior implementation. This one might be able to edge closer to 1,000 circles.
-
Here is the result from an improved version of my algorithm, producing the same 750 circles with 250k attempt cap. It now does more accurate occupied tracking, by only marking grid cells as occupied when they are actually occupied (as determined by at least one of the four corners of a grid cell being within or on the circumference of a circle). Unfortunately, it still uses a bounding box template for a circle to be placed to determine whether it can be placed. This is due to the fact that the number of placement attempts, in total, is quite high and the determination of the actual cells that would be occupied quite expensive, relatively speaking. Therefore, it is only done when a circle is placed rather than during attempts of "can it be placed here." Now at least there is more space available to place it('s bounding box). First the new times of five runs: 750 circles have been generated in 2.97 seconds 750 circles have been generated in 6.31 seconds 750 circles have been generated in 4.33 seconds 750 circles have been generated in 3.03 seconds 750 circles have been generated in 2.68 seconds Below is one of the images produced showing less wasted space, although one has to bear in mind that as each of the circles got placed, its bounding box was used to test for overlap with areas already occupied by (slightly exaggerated approximations of the locations of) existing circles. This resulted in some placement attempts being deemed invalid because the corners of the bounding box of a candidate circle overlapped existing geometry, but if the algorithm was smarter it would see that the circle itself did not. I will think about this more and see if I can come up with a way to improve on this and possibly get rid of the bounding box testing altogether, without greatly sacrificing the run time per test.
-
To really stress my implementation, I ran it again using the exact same parameters you used, except that I set the cap on the number of circles to generate to 750. Going much higher than this value would result in many failures, when the max attempts cap is limited to 250k (per random circle), before giving up. rect_bounds=RectBounds(c4d.Vector(-100, -100, 0), 200, 200) min_radius=1 max_radius=50 num_circles=750 max_circle_creation_attempts=250000 # If we can't find space for a circle after 250k attempts, give up Here are the timing of five separate runs that succeeded (they all did): 750 have been generated in 3.75 seconds 750 have been generated in 8.25 seconds 750 have been generated in 10.4 seconds 750 have been generated in 8.8 seconds 750 have been generated in 7.94 seconds ..., and here is a screenshot of one of the five versions generated: As can be seen in the above screenshot, the bounding box based algorithm I employed for collision testing is quite evident in the geometry produced, when said geometry is at this very high level of density. The inefficiencies in terms of the use of space with this algorithm manifest themselves as unused "triangular" shaped gaps that could have potentially been filled by a more efficient (and perhaps more complex/costly) space occupation tracker. This is especially noticeable around the larger circles that are present in the above sample generated image. Nevertheless, the algorithm did finish all five test attempts in a reasonable amount of time and did so successfully, within the allowed cap of 250k max attempts to randomly place a circle, before giving up and aborting.
-
I wonder how long this approach takes, time wise, compared to the approach I took using a "Battleship - the game" style peg-board approach to maintaining which logical grid cells are already occupied and disallow circle generation in those regions (that would potentially intersect other circles in that region). Here is an example of what my approach produced almost instantly, with the parameters: rect_bounds=RectBounds(c4d.Vector(-5, -5, 0), 10, 10) # I.e., width=10, height=10 generating a rect from: (-5,-5,0) to: (5,5,0) num_circles = 100 min_radius = rect_bounds.width/42 # i.e., 10/42 = 0.2381... # i.e., the circles are not allowed to have a radius smaller than this min_radius - that's cheating! max_radius = rect_bounds.width/12 # i.e., 10/12 = 0.8333... # ..., or larger than this max_radius max_circle_creation_attempts = 250000 # If we can't find space for a circle after 250k attempts, give up It took about half a second to solve, given the above parameters:
-
Yes, I was referring not to the image, but the first code excerpt Below is a complete working implementation of the algorithm I previously described, including Undo support. The added code begins where the previous code left off, except that I stripped out all of the AI generated code and comments related to it, to simplify things. I left the original comments for the code I added intact, however. It definitely could use more comments as to what each of the newly added parts does. Having said that, it correctly implements the creation of non-intersecting circles within the rectangle's boundaries using the algorithm I previously described and parameters that can be modified/tweaked within the code. Error checking at this point is very minimal, when present at all. Note that this is a fairly simplistic implementation that is far from optimal. Copy and paste it into an empty C4D Script Manager script and then click on the Execute button to try it out and of course feel free to play with the code and the various tunable generation parameters:
-
Indeed! It does and proceeds no further, but you would need to open the Console Window to actually see the error (Extensions / Console from the Main Menu or Shift-F10 [on Windows] and make sure that Python is selected from the list of various consoles on the left hand side of the Console window). Don't count on anything the Script Manager reports back (if anything at all, since in the latest C4D it reports nothing for this particular error when you click Execute). The Console Window is authoritative when errors of any kind (syntax or exception based) arise in/from Python scripts. Thank you for your kind words and appreciation! I am not sure what you mean by zero radius. I see the following: radius = random.uniform(0.1, 4.9) # Generate a random radius between 0.1 and 4.9 circle.SetRadius(radius) Now, I am not saying the rest of the code is correct, since it most definitely is not, but the circle radius part does attempt to create a radius using a random value from the closed domain [0.1, 4.9] (i.e., from 0.1 to 4.9, inclusive). Now, a comment on your Step #2: Check if it intersects with any other element (circle) This step is extremely cost prohibitive and the check for circle/"other circle" intersection must be minimized, or we have an explosion of comparisons that must be made on the order of N2 (i.e., O(N2) time complexity in Computational Complexity lingo), where N represents the number of circles that get generated. In other words, the one thing we must do our best to avoid is the intersection check between each circle and every other circle, if at all possible. There are many approaches for solving this problem. Some of these are fairly complex, some are not so complicated, although far less intuitive and more difficult to come up with. I can offer an example of one of the simpler approaches that attempts to reduce the number of comparisons necessary: I think that violating randomness with your second approach goes against the grain of the problem definition and I may be wrong but it seems that you go from generating large circles to smaller and smaller circles with the above approach. The third approach is a bit difficult for me to evaluate instantaneously, so I will have to think about it.
-
... placeholder for the rest of the corrected script, to follow ...
-
And this comic excerpt is a very accurate portrayal of what would happen if you were to try to get the scripts provided by the AI to work as request (or in this case, to work at all): This is my evaluation of the first script you provided up to the point of random circle creation. My comments are all in-line as code comments. Note that comment blocks consisting of repeated minus characters are used to box code excerpts from the original script that the AI generated. E.g.: # --------------------------------------------------------------------------- # A code excerpt from the original script would go here, likely commented out # --------------------------------------------------------------------------- Here is the starting point of the makeover of the first script with details from my analysis of what is wrong with it, as well as corrections to make it work: Modified AI script with my comments and corrections You can copy and paste the above code into the C4D Script Manager, after selecting File / New Script from the manager's menu. Be sure to either delete or overwrite any boiler plate code that gets generated for a new script with the code exactly as shown, above, or you will not be able to Execute it without errors. After pasting the code into the manager, click the Execute button that is positioned at its bottom right corner and, voila, a rectangular Spline Object called "Rectangular Spline" will be visible both on the screen and in the Object Manager. Note: Occasionally, I have noticed that the Object Manager does not get properly updated for some reason, even though the object is visible in the viewport(s). If this happens, select File / New Project from the C4D Main Menu to create a new empty project and then re-execute the above code from the Script Manager (which should not get cleared by the File/New Project command).
-
Uhm, no! No, it doesn't (know how to script, even if it's [not so little] AI life depended on it). What it does know how to do well is to "wing it" and slide by, at least unless and/or until there is serious scrutiny of (all of) the information it provides by someone reasonably knowledgeable in the subject matter. I am somewhat surprised that no (self-proclaimed) C4D Python guru has tackled (in an explicit expression of their own delusions of self-grandeur) this AI generated nonsense with a play-by-play illustrating the AI's complete and utter incompetence at providing a viable solution to the problem, as stated. What's far worse with regard to the AI's responses is the sense of complete authority and all-knowingness that it seems to convey in its choice of verbiage, almost as if what it is saying is gospel, complete, and without any fault or omission - how dare you question it further. If the answers doesn't work as described, "clearly" the fault will lie between a pair of eyes and a pair of hands and could not possibly be the generated Python scripts, themselves. There is an old Russian phrase that describes this AI's arrogant attitude: "глупая самоуверенность." Translated literally into English, the phrase means, "foolish self-confidence," (and props to Google translate for getting this one right!) although perhaps the more verbose, "foolish self-confidence stemming from ignorance," would be a better translation, since it further conveys the underlying hidden meaning that is present in the original Russian phrase. The phrase is generally and perhaps unsurprisingly used as one would expect - as an expression of the great disproportion between the air of certainty and confidence that is present within the information one is provided, when viewed within the context of the knowledge and familiarity level of the person providing it. I'll start by giving the AI a tiny bit of credit (credit is too strong of a word, maybe experimental error would be more appropriate), since there seems to be a modicum of self-doubt in its later responses when the "customer comes back for a refund or replacement for the lemon of a product that was 'sold' to them." Namely, it is its response in the form of "..., it could be that there is a problem with the scripts themselves," only to immediately back-paddle and once again blame the user with, "... or with the way they are being used." One could also interpret the phrase "scripts themselves" as a problem with how the scripts were entered, rather than the (absolute) fact that they were invalid as generated, from a functional perspective. I am not just referring to the lack of self-intersection tests, but, quite frankly, all of it - all of the crappy code that was generated! On top of that, the code was not even well-formed, syntactically. I realize that the vast majority of the above criticism was destructive in nature and did not provide much in terms of what specifically was wrong, as alluded to in the first paragraph. Therefore, in subsequent posts, let me do a full play-by-play, line-by-line of the AI code vomit that did get spewed out, starting from HappyPolygon's original request, as well as the AI's responses to the well thought-out (IMHO) subsequent requests from HappyPolygon that followed, when things did not go as planned/requested and the AI got called on its "bluff." As part of this process, I will take the original code and make changes to it, to get it to work, showing what it will take in terms of corrections to get us there as well as consider alternative approaches that the AI could have chosen to solve the problem, along the way. One of the reasons I am going through this exercise is to show how much farther this AI has to go, before it can be reasonably used even as an adjuvant (not even considering, replacement) for Software Development, as has been repeatedly touted by various forms of (ignorant) media to the (no less ignorant) masses in recent months, due to a fitting use for the previously elucidated old Russian phrase, "глупая самоуверенность." Stay tuned, as this (possible) novel will likely wind up being split up it into multiple posts and heck, if you are new to Python or C4D Python you may pick some up along the way, as an added bonus.
-
Update: The bug is still a bug, but I found the root cause and it is far more subtle (and specific) than previously described. I will also include very easy steps to reproduce it, at the bottom of the post. I am not sure how, but my perspective camera was set to be a Spherical Camera with the following settings: So, the problem is limited to the use of a Spherical camera in a Perspective View and can easily be reproduced using these steps: 1. In a Perspective viewport, enable the default Camera Object to be a Spherical Camera, with the settings shown above (which should be the default settings for a Spherical Camera). 1. Create a 10 cm x 10 cm Plane object with: => 2 Width segments => 2 Height Segments => Orientation +Y 2. Make this Plane object editable 3. Make sure global snap settings are disabled 3. Using the Line Cut tool with its tool properties set to their default values: ..., make a cut from any of the four corners of the editable polygonal plane to the central point shared by the four squares that make up the object, so as to bisect one of the four component squares into two triangles. Due to some sort of issue, when this cut is performed while viewed through a Spherical Perspective Camera, it will result in a bad cut. If you "accidentally" get a good cut, undo and try cutting from one of the other three corners to the central point and/or slightly rotating the camera. In the end, this bug is far more isolated than I thought at first, seemingly, as of right now, only effecting a Spherical Camera in Perspective mode. It is still however a bona-fide bug, although far less critical in severity and impact than I initially thought.
-
Important Update: The issue only impacts a seemingly seldom used camera type in Perspective mode. This issue is far more isolated and therefore less serious than I initially thought. As far as I can tell as of right now, it only affects the use of a Spherical Camera in a Perspective View, which is not the default setting. For further details, read the following update to the thread presenting the nature of the issue: I don't want to derail this thread and take it off-topic. Therefore, I have created a new thread (and did so in the appropriate [Cinema 4D] sub-forum of the site, rather than this sub-forum which is intended for posts associated with News) to both describe and illustrate the issues that were encountered while using the Line Cut tool. You can view the full info by clicking on the link/image, below, to view the new post:
-
Very Important Read the following update to this thread, before reading any further into this post: It appears that there are serious bugs in the Line Cut tool in the current version when the tool is used in a Perspective View to make cuts that connect points across multiple polygons. I have not noticed this type of (mis)behavior in prior versions, assuming that the tool was used properly and that the geometry it was used on was not malformed, of course. The following scene segment is a relatively minimal excerpt from a more complex scene that will be used to demonstrate an instance of the issues encountered while using the tool in the context described in the first paragraph. Starting State and Background Info The intent of the following scene object fragment is for it to be extended using four way Symmetry (i.e., along X and Z axes) to ultimately create a solid shape (once a ceiling and floor also get added). However, as you can see illustrated by the Mesh Checker, we presently have some triangles and "ugly" poles that are present in the starting geometry. To take the first step towards removing these, line cuts around the poles will be made to split up some polygons and connect the SDS support edges of the top vertical column quarter-portion with the foundational column quarter-portion. I should add that the ledge that connects the two columns, upper and lower (which themselves only contain polygons that are vertical [i.e., parallel to either the yz-plane or the xy-plane]), is horizontal and parallel to the ground (i.e., the xz-plane), since these properties may not be intuitive due to the perspective angle that is used. Information about the world coordinates of all points that make up the object's geometry, bearing in mind that the object's axis is located at the origin, will be shown in the images that follow. Notes: - The scene consists of a sole polygonal object named Cube.1 - We are looking at it in a Perspective Viewport (with the editing mode set to Edge mode) - All geometry that is present in the scene is shown in the above image - - There is no bad geometry present and everything that is present is shown, specifically: - No isolated points - No coincident points - No points that are very close (i.e., nearly coincident points that Optimize would remove/weld) - No partially or fully overlapping edges - No partially or fully overlapping polygons - No non-manifolds (any two polygons share at most one edge and each edge connects no more than two polygons) - No "bad" (i.e., degenerate) polygons - No N-Gons are present (yet!) - There are no rear facing polygons in this scene - all normals are properly aligned and face in a direction that is at least partially towards the camera. I did not want to obfuscate the image and make it more complex by enabling vertex/polygon normal line visibility and in any case, this information, at least for the polygons, is implicit in the polygon point order shown in the Structure Manager. - There is no hidden geometry - "what you see is what is there." Update: I have decided to include a small image explicitly showing all point and edge normals, as well as all polygon facing directions (via color), in case this info happens to be of relevance. Since I deem this to be largely inconsequential (as long as it is as described with regard to there being to back-facing polygons), it will not be present in any other images that follow, so as not to obscure any far more relevant details that are shown in said images: Preview of Corrected Topology Before proceeding with the steps of the first cut, I would like to present as a detailed zoomed-in illustration, the final desirable corner topology that is being worked towards via the cut operations that will be performed: I should note that only the first simple cut will actually be shown, since we immediate run into the buggy behavior that is the subject of this post. Initiation of the First Line Cut I have zoomed in on the object somewhat and have also created (and activated) a Perspective Camera (which I have unimaginatively called Camera), so that I can include all of the relevant coordinate info about the viewpoint from which the object is being viewed as well as various other camera settings (e.g., zoom, field of view, etc.), as we initiate our cut. All of this info along with additional info that include the Cube.1 object's geometry as reported by the Structure Manager will be superimposed on the viewport image that follows. We will start with a a simple linear cut to connect Point #14 to Point #10 (these points have been labeled in the image below), using the best tool for the job, the Line Cut tool. The initiation of the cut is shown in the following image where we have performed the following set of steps: 1. In a Perspective View, we select the Cube.1 object and switch the scene editing mode to Edge Mode 2. Using the Brush Selection tool, points #14 and #10 are selected (optional step to match the image shown below). There are no other selections present 3. We then set the active tool to be the Line Cut tool (i.e., <k>-<k> shortcut combo) Note: Scene-wide snaps are presently disabled (see the partial snapshot of the toolbar in the top right corner of the image), so as not to conflict with the Auto Snap functionality of the Line Cut tool, which will be enabled The remaining additional Line Cut tool properties presently set are as shown in the image (bottom right corner - "Line cut settings:") 5. We click on Point #14 to initiate the cut and subsequently click on Point #10 to complete the cut. Note: I will show multiple Snapshots of the Structure Manager both pre-cut and post-cut, so that all of the details with regard to the Cube.1 object's make-up are explicit. Because the Line Cut tool has been set to Single Line mode, no further actions will be necessary and our cut operation is complete. Resulting Aberrant Geometry As we complete the aforementioned cut operation, we see the following erroneous result: ..., and there in lies the issue. The Line Cut tool did not stop its cut at Point #10, as instructed. It performed some additional seemingly random cutting along the path of the cut line. It behaved in a way that is similar (but not identical) to what would have happened if the Infinite Cut property of the Line Cut tool had been set. Furthermore, if we perform the exact same cut, but with the Single Line property unchecked, causing the Infinite Cut property to be grayed out since this type of cut would no longer be possible or applicable, we get exactly the same cut and geometry as shown in the above image (i.e., this has nothing to do with Infinite Cut as far as I can tell, it just looks similar in that the cut continues along the direction of cut, erroneously cutting other polygons of the object [and then some!]). I will be somewhat busy for the next several days as the year comes to a close, but feel free to ask any questions regarding state or steps not present in the above description. I have done my best to be quite thorough and omit no information that may be of relevance in troubleshooting the bug. Although I cannot include the original scene file (or even the excerpt shown), I believe all info need to reproduce this is in the body of this text. If I have omitted anything of value or relevance, I will do my best to provide it as an update to this message. Addendum I decided to include an additional test I performed using the more complex geometry that was shown previously as the final desired topology for the corner. The test was to do a simple cut of one of the quads present near the middle of the object, simply to cut it in half (i.e., into two triangles). Specifically, I decided to try to connect the following selected pair of points with a single cut through the single polygon that they are the corners of, cutting from the top right point to the bottom left using the exact same Line Cut settings that were previously used. Let's start with a view showing the entire topological structure of the object, both with and without a Subdivision Surface generator applied to it (Note: The object has a bottom face that is obscured by the view. It looks similar, topologically, to its top face. Also of note is that the object has no back face (and lacks the corresponding control edges along its farthest [i.e., maximal Z+] edges), since it is actually the front half of an object that is intended to be used with an xy-aligned Symmetry Generator to form a complete solid object): Original: , with SDS: The initial state showing the two points that are the bounds of the cut being performed: Just before clicking on the bottom left point to complete the cut, being very careful not to move the mouse during either click, so as not to lose Line Cut tool vertex snap: : The resulting mess, post cut (shown higher-res for added detail of the resulting topology): In the next image, I zoomed out to show the entirety of the object once again, but this time, post cut. (Note: The obscured bottom face was not altered in any way by the cut): So, there you have it - don't know what else to add. Updates and Clarifications Reserved - will be entered into this top post based on replies, as they arise...
-
The Line Cut tool when used to cut across several polygons in a Perspective view is very broken in the latest version of C4D 2023! I don't know in specifically which release the bug "was born," but I am going to post more details with a minimal test scene to demonstrate the bug(s), in a little bit.
-
So what abour your Patreon? Will you be keeping that up? I'd hate to lose all of the tips accumulated there over the years! Or, perhaps you could combine it all into a PDF or something for us to download?
-
Good to know that this is a known bug in Cinema 4D 2023.1. Also, whether the Correction deformer is inside of a Null, a child of the parametric object, or even deleted after the fact, it doesn't much matter for this case. It is only the Polygonal Selection Tag that gets placed on the parametric object that has any effect on subsequent material tags. The Correction deformer literally does nothing after the Polygon Selection Tag is transferred out of it and can be deleted.
-
It used to be that you could use a Null to group a Correction deformer with a parametric object like a Cube. Then you could use the Correction deformer to create a Polygon Selection Tag (which we will call s1) of some of the Cube object's polygons which you could then drag and drop on your Cube to be able to select just those polygons for subsequent Material Tags. You could then drop a material on the Cube using a Material Tag and limit its effect to just the selected polygons associated with the previously created Polygon Selection Tag by setting the Material Tag's Selection property to s1 (i.e., the name previously given to the Polygon Selection Tag). This no longer appears to work, at least in Cinema 4D 2023, but I don't know how early in the version list it stopped working. In other words, if a Material Tag is added to the tags of a parametric object such as a Cube and it contains a name in its Selection property that correctly refers to a preceding Polygon Selection Tag on the Cube (containing a completely valid polygon selection for the Cube), the Material Tag stops having any effect, whatsoever.
-
The problem with projection is that it is linear - only using the camera looking at object direction. Often you want to project towards a central point (spherical projection) or a central axis (cylindrical projection). This is when life gets difficult with Cinema 4D, because you have a two or three axis, somewhat perspective-like projection of an object's points, or subset of points, towards a central point or line.
-
I will start the conversation by suggesting that you create a Null object as a child of your hand at whatever point you want to follow and have the object being moved track this Null rather than a point or points on your "hand." You can use xpresso to tie the position of the Null to the world position of the point on the hand. I will add that this is likely not the best approach, but it is a relatively simple one.
-
Yes, it certainly looks good, best I can tell. I wish you would have posted the actual topology, rather than the ISO version, so I can make out what's happening there. When I tried the same approach I had horrible artifacting, so the ratio of outer radius to inner radius of the starting tube, along with the size of the bar cut may play a role. In any case, I want to try Shepper's approach and see where that gets me, then compare artifacts with my solution and perhaps post the scene file if you want to give it a go with the remesher or maybe I'll give it a go. I used a Volume Builder/Mesher/Remesher combination for my attempt. Maybe, it fares better with Boole - will try.
-
This is exactly the sort of thing that the nodal system needs - intermediate level functionality that is tweakable.
-
This is intended for SDS. No use of the Boole generator and most certainly no volume builder (not even with ZRemesher based remeshing) - they are both horrible at solving difficult hard-body problems like this one, correctly, at least based on my testing). This is a misconception: - Yes, it is not ideal from a phong shading perspective and should be avoided when possible - No tearing does not have to occur. If tearing is occurring, it is because of Cinema's inability to accept a certain point ordering at the polygon level and this can often be corrected using one of two methods: - Deleting the errantly handled polygon and using the Fill Polygon Hole functionality, or in the worst case - Rotating the a, b, c, d polygon point order (but, not reversing the point order direction, since this would invert the polygon's normal), until the tearing goes away. I agree. If you look at my topological solution, especially the last two attached images, I did exactly that. With regard to ShepperOneill's method. I did not try that, but will give it a go to see if it makes things better. Unfortunately, he does not show us the final topology in that video, but at the 11:00 mark, you can, with some difficulty due to the horrible contrast between the Gouraud shaded vase and the edge lines, at least see how the topology of three of the four corners (i.e., specifically, all but the upper right corner) has been laid out: A special note to those making educational videos: Never ever ever use a Gouraud Shading (Lines) viewport Display setting to show topology to your audience! Always, and I mean almost without exception, use Constant Shading (Lines) with a good choice of colors that provides a significant amount of contrast between the colors used for the edges/points and the logical "background" on which they are shown (i.e., the color chosen for the object's surface - preferably, with the Basic/Display Color property set to Custom and a good choice of contrasting color). I'll report back after changing to Sheppard's method from my original topology with regard to artifact elimination. Actually, comparing the two, his topology is very similar to mine, with the exception of him eliminating those concave quads, so it will be easy to alter mine to match.
-
Update: Yes, this topology is intended to be used with SDS subdivision. Let me start by presenting you with an image of the sort of hole we're talking about. We want to cut the red rectangular bar out of the blue pipe object, leaving two rectangular holes (at least when viewed from the face of the pipe. Items of note: The outer surface topology is different from the inner surface topology, since the inner radius of a tube is smaller than its outer one. The yellow arrows in the scene show that only a 4 x 2 quad section of the outer surface is cut out, while the cyan arrows point to the corresponding, almost 6 x 2 quad section that would need to be cut from the inner surface. Now, given a good starting choice for the topology of the tube, based on the dimensions of the hole to be made, I have come up with the following solution, with one outer edge highlighted along with one inner edge on the opposite side of the cylinder. I have positioned the camera so that both the outer and inner topology is visible in a single image, for purposes of comparison and evaluation of what I went with to solve this: I am somewhat OK with the outer topology, even though it is not perfect at certain angles, but the inner topology is not nearly as clean, producing far more visible artifacts under subdivision, regardless of the level set. Any comments or ideas are welcome. I should immediately note that the technique to start from a box, cut out a hole, and then use a bend modifier to make a tube out of it, would not work here. This is because the technique would produce an inner hole that is smaller in size than the outer hole, with the hole itself shaped like a pyramid, getting smaller from outside to inside. I will add two more images, slightly zoomed out, so that surrounding topology is more visible. This is the view from the outside showing the topology of the outer surface (note that the far half of the tube is hidden in this image, so as not to cause confusion): And here is a view showing the asymmetry of the inner topology, viewed from inside the tube, looking out:
-
This is a classic case of knee/elbow joint overlap issues that can be solved by a combination of C4D bones and skin in combination with vertex weighting and the proper topology to allow for good realistic bends. There are a few youtube videos available that illustrate the technique.